JavaScript is all about dynamic stuff and dealing with dynamic CSS changes is just one of the scenarios where JavaScript is exactly what we need.
With JavaScript, we are able to set CSS styles for one or multiple elements in the DOM, modify them, remove them or even change the whole stylesheet for all your page.
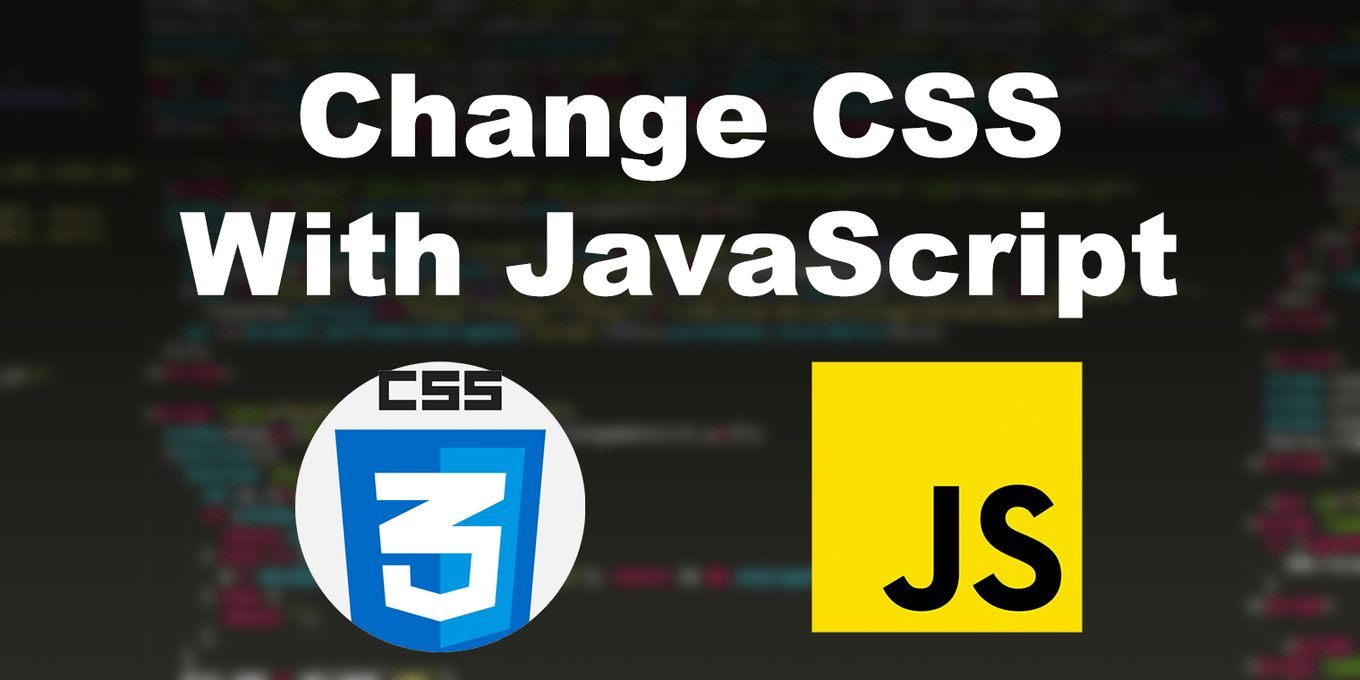
Let’s get into the different ways we can do achieve this:
1. Change CSS inline properties with JavaScript
Setting individual styles directly from JavaScript is one of the most common scenarios when dealing with dynamic CSS styles.
This way allows you to change the CSS styles for one or multiple elements present in the DOM.
All you have to do is:
- Query the element present in the DOM.
- And set the style or styles for it one by one.
const element = document.querySelector('.demo');
element.style.backgroundColor = 'red';
Code language: JavaScript (javascript)
Notice that when setting the CSS styles using the
style
property you have to write the CSS properties in camelcase. Instead of using a dash-
to separate the words you will have to write in capitals the first letter of each word. (backgroundColor
,fontSize
)
If you execute this code just like this, you’ll notice the change takes place on page load.
If you want to change the CSS styles dynamically you’ll have to attach this portion of code to some event.
For example, if you want to change the styles of your element when clicking on a button, then you have to first listen to the click
event and attach a function containing the previous code.
Here’s an example:
const button = document.querySelector('button');
button.addEventListener('click', () => {
const element = document.querySelector('.demo');
element.style.backgroundColor = 'red';
});
Code language: JavaScript (javascript)
Note that when you apply specific styles using JavaScript, these styles have precedence over the styles applied externally on a stylesheet and even to the ones applied inline, in the HTML element itself.
.demo{
color: red;
}
Code language: CSS (css)
<!-- Element with inline styles declared in the HTML -->
<div class="demo" style="color: blue;">Demo</div>
Code language: HTML, XML (xml)
In this case, for instance, we have an element with an inline style providing it with a yellow background.
If we now set the CSS color
property to green
using JavaScript, then our element will get a green
color. It will overwrite the inline style and the style applied on the external CSS stylesheet.
2. Set Multiple CSS Styles At The Same Time
Imagine you have to apply, let’s say 5 or 10 styles for a single element.
You can go one by one and have something like the following:
element.style.display = 'block';
element.style.width = '100px';
element.style.backgroundColor = 'red';
element.style.border = '2px';
element.style.fontSize = '12px';
element.style.color = 'white';
element.style.margin = '20px';
element.style.paddingLeft = '10px';
element.style.paddingBottom = '10px';
element.style.lineHeight = '13px';
Code language: JavaScript (javascript)
But perhaps you are looking for a “smarter” way to change them all at the same time without so much code repetition.
If that’s the case, then I have good news for you!
You can actually pass a string
value to the cssText
property to set multiple CSS styles at once.
Even better, we can use template literals kind of strings to keep them separate in different lines as you do in your stylesheets.
// Defining all our CSS styles
const myStyles = `
display: block;
width: 80%;
background-color: red;
border: 2px;
font-size: 5em;
color: white;
margin: 20px;
padding-left: 10px;
padding-bottom: 10px;
border: 2px solid black;
`;
const element = document.querySelector('.demo');
element.style.cssText = myStyles;
Code language: JavaScript (javascript)
If you are getting into JavaScript you might want to check What’s The Best Way To Learn JavaScript.
2. Change CSS class in JavaScript
<div class="demo">Hello</div>
Code language: HTML, XML (xml)
// Adding a CSS class name to an element
const element = document.querySelector('.demo');
element.classList.add('new-class');
Code language: JavaScript (javascript)
.new-class{
background: red;
}
Code language: CSS (css)
In the same way, you can also remove certain classes by using classList.remove
or even toggle them when using classList.toggle
.
Here’s an example:
// Removing an existing class from an element
const element = document.querySelector('.demo');
element.classList.removeClass('.new-class');
Code language: JavaScript (javascript)
// Toggling a class from an element
const element = document.querySelector('.demo');
element.classList.toggleClass('.new-class');
Code language: JavaScript (javascript)
3. Change CSS stylesheets dynamically
Let’s say that now you do not want to add inline styles to an element or apply a class to it. Instead, you want to apply a change at the stylesheet level. Why?
There are a couple of reasons:
-
You might want to apply the change to all elements with a certain selector. But not just to the elements present right now on the HTML, but also to all future ones that will be added dynamically later on.
-
There might be a huge amount of elements sharing the selector you want to apply changes to. Imagine 500 elements sharing your selector, or even 1K, 5K, 10K. Having to select all those elements using JavaScript and then loop through them to change their style (or add a class) will be EXTREMELY slow.
Here’s where modifying the CSS stylesheet directly will come on handy.
You can select the stylesheets of a document by using document.styleSheets
. If you know that the stylesheet you want to modify is the second one, you can get it by using document.styleSheets[2]
.
Then, you would loop through all its rules and get the one you need to modify. In this case, if you want to modify the .element
class, you can compare each of the rules’ selector with .element
.
Here’s the code:
// Getting the stylesheet
const stylesheet = document.styleSheets[2];
let elementRules;
// looping through all its rules and getting your rule
for(let i = 0; i < stylesheet.cssRules.length; i++) {
if(stylesheet.cssRules[i].selectorText === '.element') {
elementRules = stylesheet.cssRules[i];
}
}
// modifying the rule in the stylesheet
elementRules.style.setProperty('background', 'blue');
Code language: JavaScript (javascript)
And here is the Codepen example that you can play with:
4. Append And Remove CSS stylesheets dynamically
In some cases, we might want to just append a whole new stylesheet or even replace an existing one.
The reason for it might be:
-
You have a web application that supports multiple themes and allows the user to change them dynamically.
-
You might want to package a component in a single JS file instead of having to include both files, the JS and the CSS one.
The way this works is quite simple:
- 1- We write the content for our new stylesheet using template literals.
- 2- We select the
head
element of the page to append our new stylesheet. - 3- We create a stylesheet element using
document.createElement("style")
- 4- We append our stylesheet content to the new style element by using
document.createTextNode
andappendChild
.
Here’s the code:
const button = document.querySelector("button");
// The content of the stylesheet
const styleSheetContent = `
.demo{
background:red;
}
`;
// Attaching the change to a click event in a button
button.addEventListener("click", () => {
appendStyleSheet("demo", styleSheetContent);
});
// Appends CSS content to the head of the site
function appendStyleSheet(id, content) {
if (!document.querySelector("#" + id)) {
var head = document.head || document.getElementsByTagName("head")[0];
console.log(head);
head.appendChild(createStyleElement(id, content));
}
}
// Creates the style element
function createStyleElement(id, content) {
var style = document.createElement("style");
style.type = "text/css";
style.id = id;
if (style.styleSheet) {
style.styleSheet.cssText = content;
} else {
style.appendChild(document.createTextNode(content));
}
return style;
}
Code language: JavaScript (javascript)
And here is the Codepen example so you can test it by yourself and modify a few things to your needs:
5. Overwrite CSS !important
style with JavaScript
We all know the rule: “Avoid using !important”. But hey! Sometimes it’s really necessary or it is just out of our control.
The !important
priority property makes sure that such style will be overwriting any inline declared style (written in the HTML element itself) as well as any previously declared rule that applies to your element.
So… what if we need to overwrite the style that was previously declared using !important
?
Imagine we have our element:
<div class="demo">Demo</div>
Code language: HTML, XML (xml)
And the stylesheet using the !important
rule like so:
.demo{
background-color: yellow !important;
}
Code language: CSS (css)
All you have to do is apply the priority
parameter on the setProperty
function that JavaScript provides us:
el.style.setProperty(property, value, priority);
Code language: JavaScript (javascript)
Here’s an example:
var el = document.querySelector('.demo');
el.style.setProperty('background-color', 'red', 'important');
Code language: JavaScript (javascript)
Notice how when using
setProperty
we specify the CSS properties exactly in the same way we do in our stylesheet. Using a dash-
to separate the words. (background-color
,font-size
)