Bruce Lee once said, “Until you have mastered anchor links in HTML and CSS, you have not mastered front end development.“
OK, I’m kidding, Bruce Lee didn’t say that. But it’s still kinda true. Anchor links (URL anchors) are often the first think aspiring devs learn to use – but there’s more to learn than simply hyperlinking from one page to another. So let us begin our path to mastery…
What Is an Anchor Link in HTML?
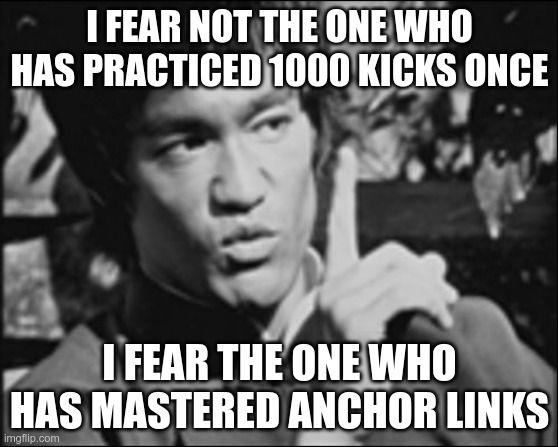
When we talk about the anchor link, we mean the <a>
HTML element. As you know, you mainly use it to hyperlink to other webpages, but you can also use it to link to email addresses, phone numbers, or even files on your local hard drive.
So why is called an ‘anchor’, and not a ‘link’ like a normal person would call it?
Well, it’s because it was originally invented as a way of hopping between different parts of the same document (internal linking in HTML) You’d use the <a>
tag not only to create the link, but also to identify the part of the page you’re linking to. Just as a real anchor stops a ship from moving, the anchor tag stopped the viewport from moving.
Nowadays, you don’t need to use the a
tag as a ‘stopping point’ – you can if you want, but you can also link to any element with an id
attribute (more on this later).
How To Add The URL Anchor in HTML
The key attribute of the anchor element is href
. You use this to state where you want the link goes:
<a href="https://alvarotrigo.com/">AlvaroTrigo.com</a>
Code language: HTML, XML (xml)
The text between the opening <a>
and closing </a>
tags is the text that people actually click – known as the anchor text.
Below are a few anchor link (URL anchor) HTML examples:
How To Link To a Specific Part Of a HTML Page?
The above examples create an URL anchor to a different web page. However as I mentioned earlier, you can also use URL anchors for internal linking in HTML pages.
To do that, you just add an id
attribute to the element you want to link to (which would usually be a header, but can be anything), and use that in the href
attribute of the anchor:
<a href="#another-section">I'm linking from here</a>
<h2 id="another-section">I'm linking to here</h2>
Code language: HTML, XML (xml)
Note that you need to put a #
in the anchor’s href
, but not in the target’s id
.
Below is a internal linking example so you can see how it looks in action:
Anchor Link To Top Of Page
OK, this works as intended. But what if our visitor wants to get back to the top? Should we just let them scroll all the way back up? We could… but let’s make it easy for them, and add another anchor link taking them to the top of the page.
To do this, we use the exact same process as before. We just need to find the highest element on our page, the text-area
div
in this case, and add an id
to it:
<div class="text-area" id="top">
Code language: HTML, XML (xml)
Then we just link to it as normal:
<a href="#top">Back to top</a>
Code language: HTML, XML (xml)
We get this:
Anchor Link Smooth Scroll
At the moment, the link just teleports people to the new location. It would be cool if instead, the page scrolled gradually down to its destination.
Not only is that possible, but it’s also pretty easy to achieve with jQuery:
You can find detailed instructions on how to do this here: jQuery smooth scroll.
Anchor Link Offset CSS
One thing though. Notice how when we scroll down the lower part of the page, we end up with no gap between our destination and the top of the viewport? It’s completely flush.
This doesn’t look very nice, so we should add a little offset above our destination, to give it a little breathing room. To do that, we can just this nice trick from Chris Coyier:
#lorem-ipsum::before {
display: block;
content: " ";
margin-top: -20px;
height: 20px;
visibility: hidden;
pointer-events: none;
}
Code language: CSS (css)
The ::before
is a pseudo-element, it will be the first child element of the thing you attach it to, in this case our destination header with the id
of #lorem-ipsum
.
By giving the psuedo-element a height
, and making it a block
element, we ensure that any sibling elements will appear on a new line below it. By setting a negative top margin, we shift it up by the same amount, which cancels out the height so our display won’t be affected. However… as far as the <a>
is concerned, that height still exists! So the target of the link is actually 20px
above the element.
Told you it was a trick!
URL Anchor CSS – Styling Your Links
OK – you can make your links do something. Now let’s think about the visual aspect. How can you change the appearance of anchor links?
Easy, just select the a
element in your CSS, and add any style you want. For example, the default anchor link colour is blue, or #0000ee
If you wanted to change that, you’d just use:
a {
color: #ee7700;
}
Code language: CSS (css)
This would give you orange links.
Here are a few other common ways you can customise your anchor links with CSS…
URL Anchor Underline CSS
Many sites get rid of the underline in their anchor links. I can see the logic here – I think every internet user knows that different coloured text is usually a link, and many people think links look better without the underline.
In CSS, you underline (or not) using the text-decoration
property, so we can just select the a
element and set that to none
:
a {
text-decoration: none;
}
Code language: CSS (css)
Let’s try it and see how it looks:
What do you think? Better, or worse? I’ll leave you to make that call on your own projects. Personally, I think the text looks cleaner without it… but I also think the underline is useful. It removes all confusion – “this is a link, 100% for sure, no question about it”. I wonder if there’s a middle ground here…
Anchor Link Hover CSS
If we want, we can target our anchor link CSS to take effect only when the visitor hovers over the link. It’s easy, just use a:hover
in the CSS.
So if you want to remove the underline from the link, but have it pop back on when people hover over it, you’d use this:
a {
text-decoration: none;
}
a:hover {
text-decoration: underline;
}
Code language: CSS (css)
The result:
OK I like it! step-by-step our page is coming together.
URL anchor Visited Color
Earlier we talked about changing the colour of the link.
But as you know, links change to a different colour if you’ve visited that destination before. You’ll have seen it in our example above – when you go back to the top, the original link is now purple – #551A8B
, to be precise. That’s the default colour for visited links.
If you want to change that, just use a:visited
:
a:visited {
color: #7B0706;
}
Code language: CSS (css)
This would make it brown.
Make an Image Into a Link In HTML
Your anchor text doesn’t have to be text. It can be any element at all!
It’s quite common to use an image as a link – for example to point to a full-size version of the image. So how do you link up an image? Easy, just put your <img>
element inside the <a>
:
<a href="#top" id="to-top"><img src="https://i.imgur.com/LOUIXcd.jpg" alt="Back to top" /></a>
Code language: HTML, XML (xml)
The cool thing here is, if the image link breaks, the alt text will appear. So the fallback is just an ordinary, fully functioning text link. Here’s an example:
Anchor Link Padding
To make your anchor links even more visible to your visitors, you might want to add a little padding around them. And your first thought, I imagine, would be to do this:
a {
padding: 10px;
}
Code language: CSS (css)
If you do this, you’ll find that the padding only appears to the left and right of the anchor link, like this:
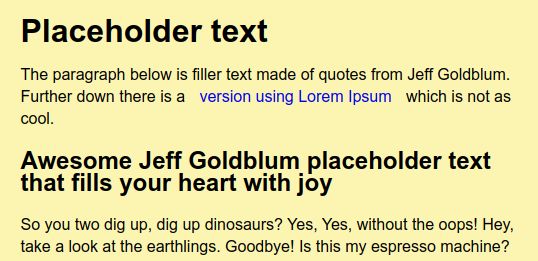
This happens because <a>
is an inline element, and all inline elements behave in this way. If that’s what you want, great! You’re done!
But if you want the padding to appear on all four sides of the anchor, you just need to make it an inline-block
element:
a {
display: inline-block;
padding: 10px;
}
Code language: CSS (css)
This applies to all inline elements, so it’s a handy thing to know about. The result is shown below – effectively, it increases the line-height
of any line that a link is on:
Nice Work Improving Your Anchor Link Skills!
OK, +100 XP for you – you now know how to create and style your anchor links!
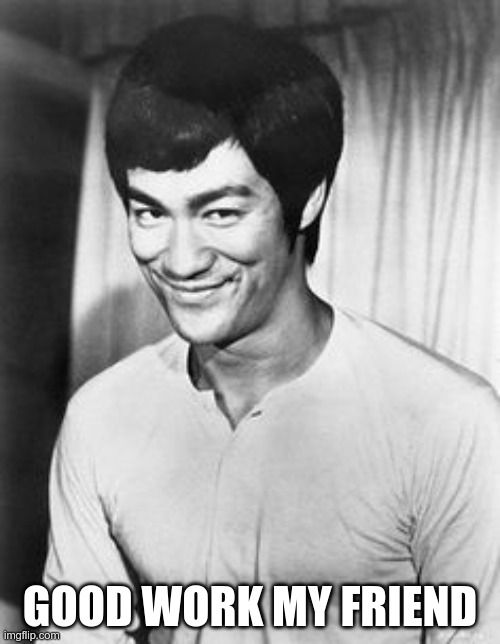
So the question now is, what’s the next step on your journey? Here’s an idea… why not try to create a full-page site? You know the type I mean – where the web page is broken into full-page segments, and you scroll down to move between them. They are commonly used by design studios and big name brands, and your portfolio will look awesome (like these ones) if you have a few of these on them.
But aren’t they really hard to make? Well, they can be, if you decide to make one from scratch. But there’s an easier way – use a JS library called fullPage.js.
fullPage.js is an easy-to-use, plug-and-play solution for making full-page sites. There are loads of different backgrounds, transition effects, and animations you can active really easily. Another cool thing is that fullPage integrates perfectly with WordPress – a lot of your potential clients (or potential employer’s clients) will use WordPress, so having a way to make your work stand out from the pack will be a huge plus. And fullPage is that way!
Take a look: fullPage.js